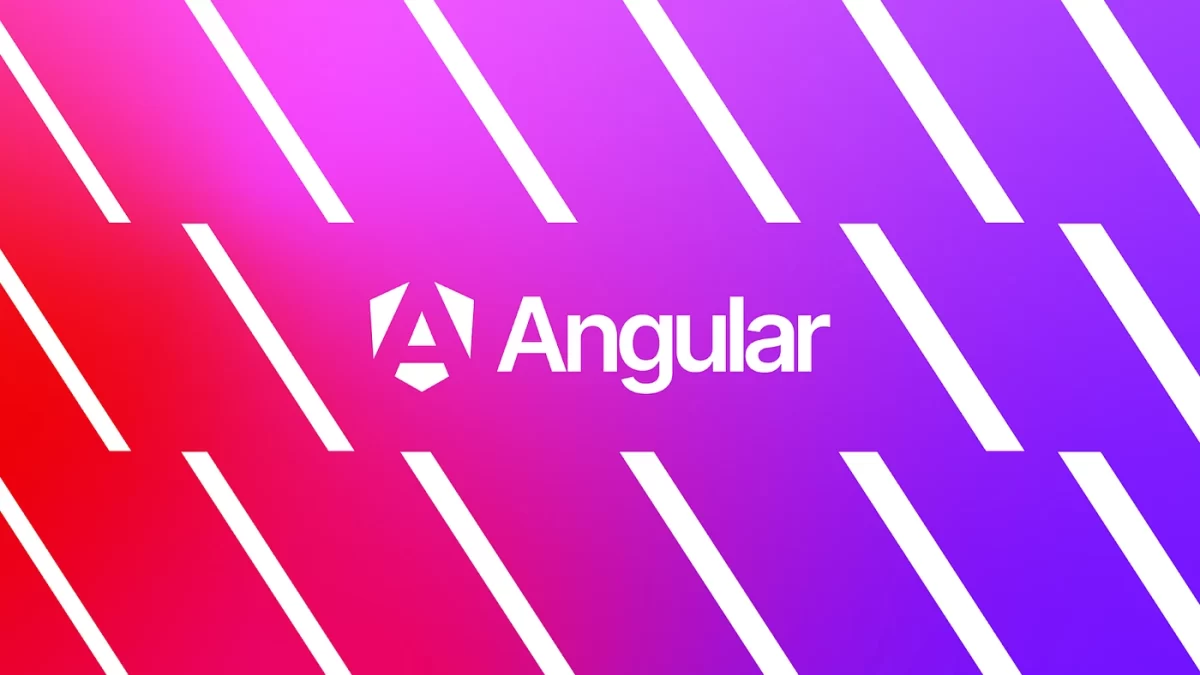
What is Data Binding in Angular?
Natan Ferreira
- 0
- 158
It’s a way of communication between TypeScript code and HTML template.
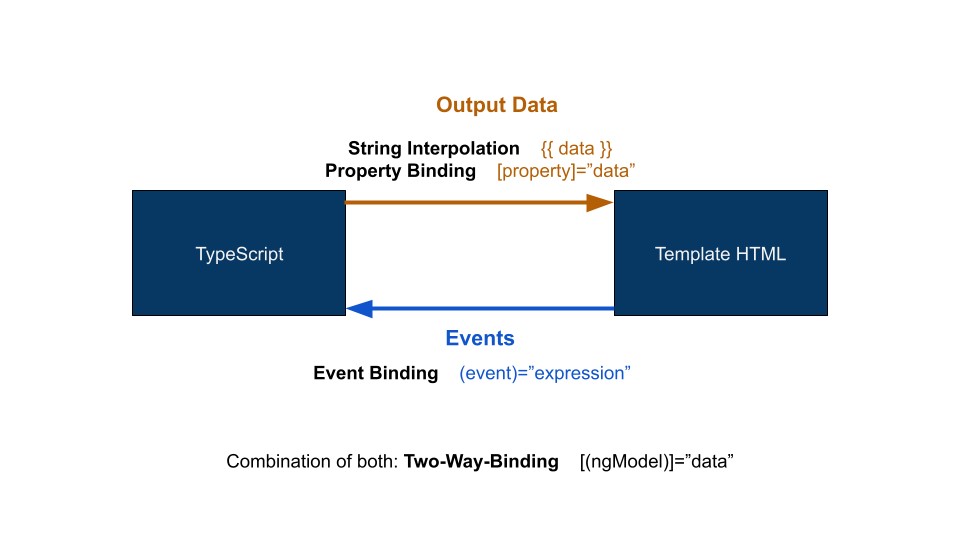
Let’s see an example below. I created a component called “user”.
import { Component } from '@angular/core';
@Component({
selector: 'app-user',
templateUrl: './user.component.html',
styleUrls: ['./user.component.css']
})
export class UserComponent {
username: string = "";
onResetUsername() {
this.username = "";
}
}
We have a variable that stores the value of the “username” and a method that resets this value, it’s a method triggered by a click event in the HTML template.
<div>
<input type="text" [(ngModel)]="username">
<button [disabled]="username === ''" (click)="onResetUsername()">Reset</button>
</div>
<div>
<h2>Welcome {{ username }}!</h2>
</div>
In the HTML template, we have examples of Property Binding, String Interpolation, Event Binding, and Two-Way Binding. Let’s look at each of them.
Property Binding
[disabled]=”username === ””
Needs to receive a true or false value, so we have this verification. It’s something that comes from the TypeScript code to the HTML template. If the value provided is true, the button is disabled.
String Interpolation
{{ username }}
Displays the value of the “username” variable.
Event Binding
(click)=”onResetUsername()”
When clicking the button, the event occurs and calls the onResetUsername()
method, which is defined in the TypeScript code. Thus, the event flows from the HTML template to the TypeScript code.
Two-Way-Binding
[(ngModel)]=”username”
It’s the combination of Property Binding and Event Binding, which means it works in both directions.
Don’t forget to use FormsModule, we need it for ngModel to work.
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { UserComponent } from './user/user.component';
import { FormsModule } from '@angular/forms';
@NgModule({
declarations: [
AppComponent,
UserComponent
],
imports: [
FormsModule,
BrowserModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Let’s see the project running:
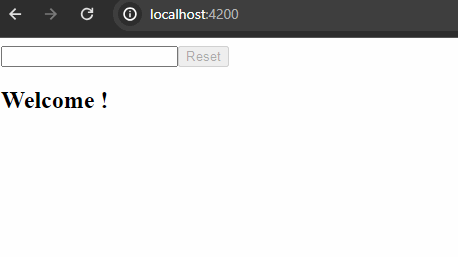
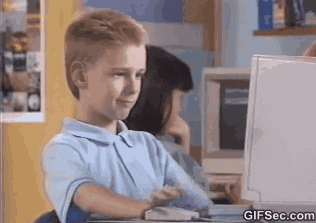
Author
-
I am a seasoned Full Stack Software Developer with 8+ years of experience, including 6+ years specializing in Java with Spring and Quarkus. My core expertise lies in developing robust RESTful APIs integrated with Cosmos Db, MySQL, and cloud platforms like Azure and AWS. I have extensive experience designing and implementing microservices architectures, ensuring performance and reliability for high-traffic systems. In addition to backend development, I have experience with Angular to build user-friendly interfaces, leveraging my postgraduate degree in frontend web development to deliver seamless and responsive user experiences. My dedication to clean and secure code led me to present best practices to my company and clients, using tools like Sonar to ensure code quality and security. I am a critical thinker, problem solver, and team player, thriving in collaborative environments while tackling complex challenges. Beyond development, I share knowledge through my blog, NatanCode, where I write about Java, Spring, Quarkus, databases, and frontend development. My passion for learning and delivering innovative solutions drives me to excel in every project I undertake.