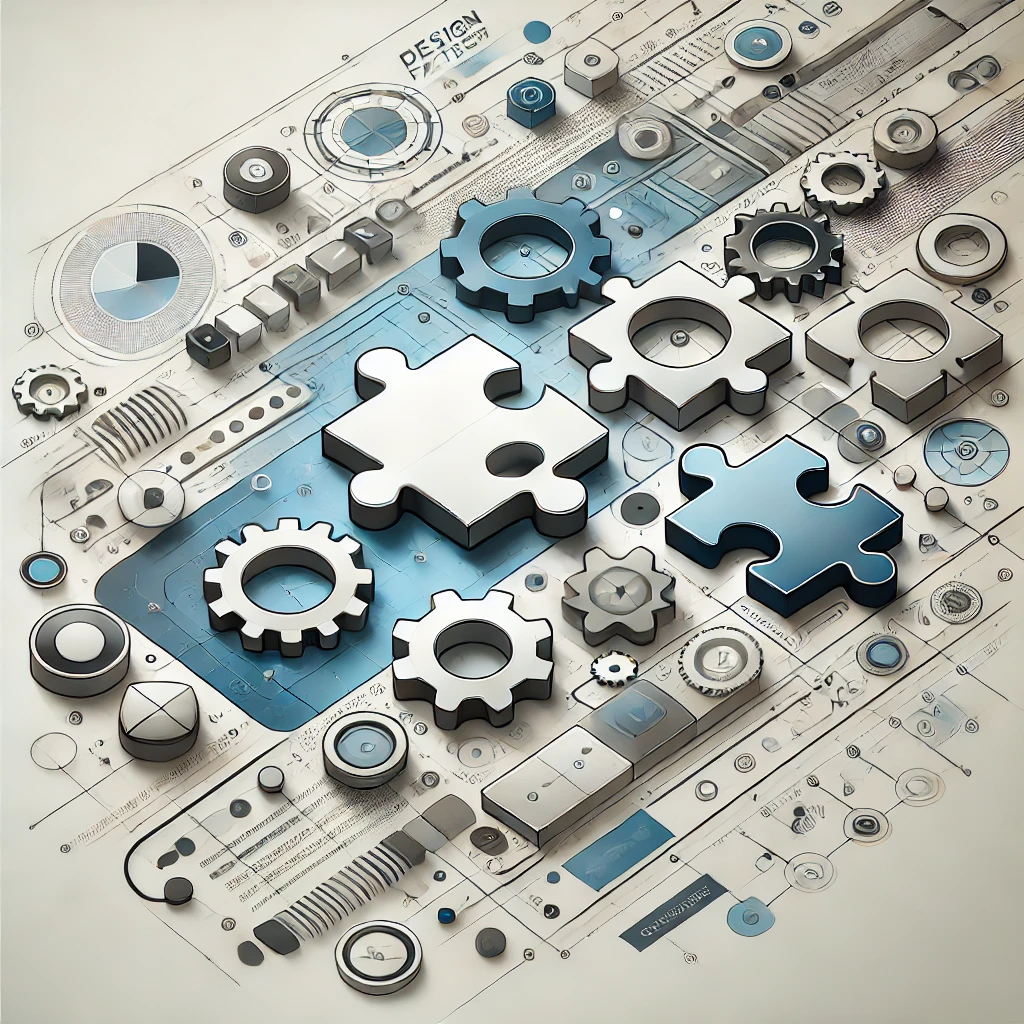
What is Factory Method?
Natan Ferreira
- 0
- 66
The Factory Method is a creational design pattern that provides an interface for creating objects in a superclass but allows subclasses to alter the type of objects that will be created.
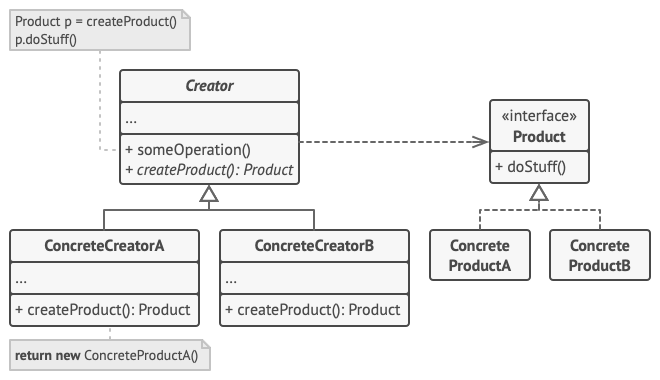
In addition to the use of polymorphism, it is possible to create either ProductA or ProductB, depending on which factory is being used. The client does not have the responsibility of creating the products; the factories handle that.
Hands on
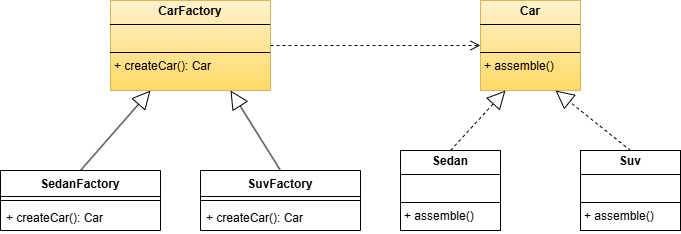
Let’s start by specifying the products.
Abstract Product
public interface Car {
void assemble();
}
Concrete Products
public class Sedan implements Car {
@Override
public void assemble() {
System.out.println("Assembling a Sedan");
}
}
public class Suv implements Car {
@Override
public void assemble() {
System.out.println("Assembling an SUV");
}
}
Now let’s specify the factories.
Abstract Factory
public abstract class CarFactory {
public abstract Car createCar();
}
It contains the createCar method, which will be used by the concrete factories, allowing us to create the concrete products.
Concrete Factories
public class SedanFactory extends CarFactory {
@Override
public Car createCar() {
return new Sedan();
}
}
public class SuvFactory extends CarFactory {
@Override
public Car createCar() {
return new Suv();
}
}
Each concrete factory returns a different concrete product. We use polymorphism, making the code more flexible.
Client
CarFactory sedanFactory = new SedanFactory();
Car sedan = sedanFactory.createCar();
sedan.assemble();
CarFactory suvFactory = new SuvFactory();
Car suvCar = suvFactory.createCar();
suvCar.assemble();
This way, the client doesn’t need to create the concrete product; the Factory holds this responsibility.
This is a flexible way to create products. If it becomes necessary to create a different type of car, we can simply add this new concrete product and create a factory for it, ensuring that the existing classes do not need to be modified.
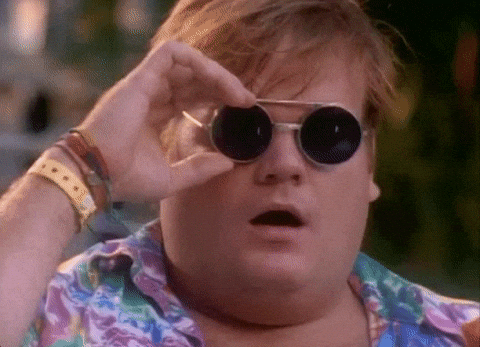
Author
-
I am a seasoned Full Stack Software Developer with 8+ years of experience, including 6+ years specializing in Java with Spring and Quarkus. My core expertise lies in developing robust RESTful APIs integrated with Cosmos Db, MySQL, and cloud platforms like Azure and AWS. I have extensive experience designing and implementing microservices architectures, ensuring performance and reliability for high-traffic systems. In addition to backend development, I have experience with Angular to build user-friendly interfaces, leveraging my postgraduate degree in frontend web development to deliver seamless and responsive user experiences. My dedication to clean and secure code led me to present best practices to my company and clients, using tools like Sonar to ensure code quality and security. I am a critical thinker, problem solver, and team player, thriving in collaborative environments while tackling complex challenges. Beyond development, I share knowledge through my blog, NatanCode, where I write about Java, Spring, Quarkus, databases, and frontend development. My passion for learning and delivering innovative solutions drives me to excel in every project I undertake.