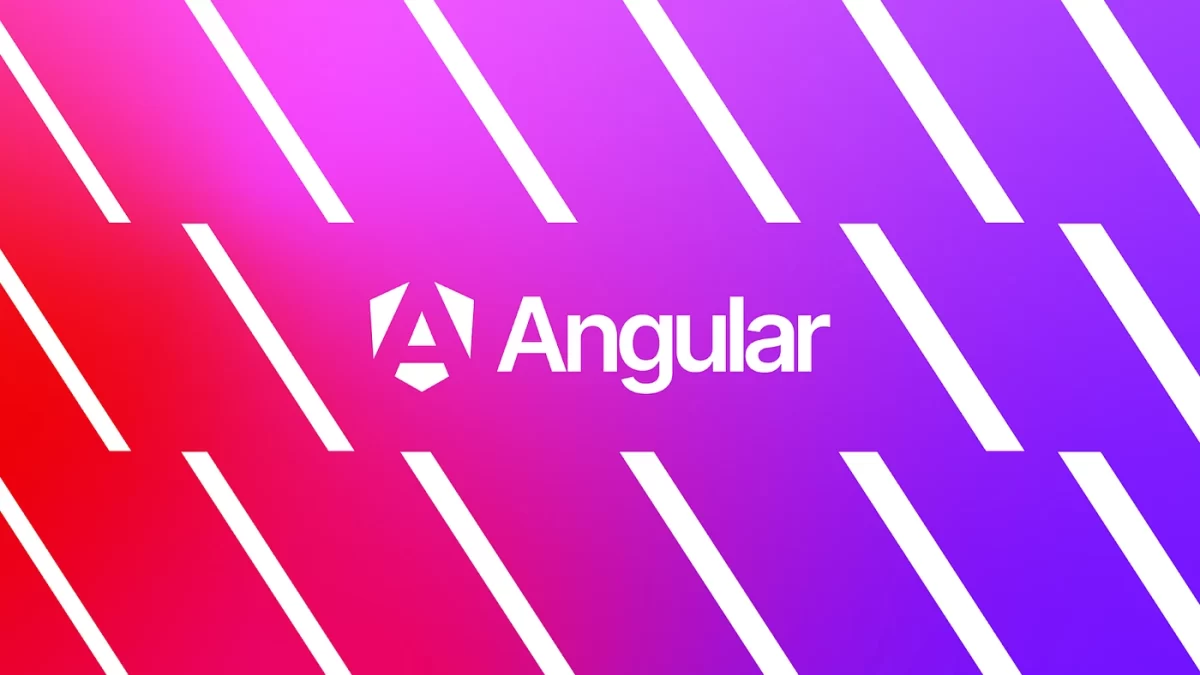
How does work New Control Flow Syntax in Angular 17 ?
Natan Ferreira
- 0
- 226
Angular 17 has undergone significant modernization, introducing new syntax, and features, and achieving better performance. Notably, they made substantial transformations. In this post, we will focus on New Control Flow Syntax.
The Angular 17 introduced the @if, @for, and @switch syntax. Let’s explore each one.
@if block conditionally
The @if block conditionally displays its content when its condition expression is truthy. Let’s see an example:
<div id="if">
<h2>If-Block</h2>
@if (a > b) {
{{a}} is greater than {{b}}
} @else if (b > a) {
{{a}} is less than {{b}}
} @else {
{{a}} is equal to {{b}}
}
</div>
a: number = 10;
b: number = 20;
The result is:
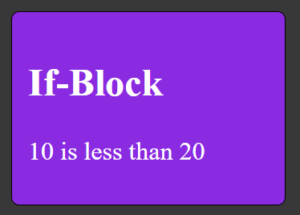
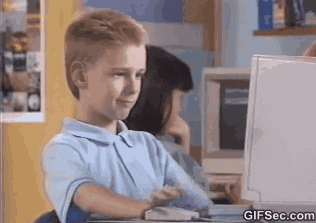
I copied the example below from the documentation, which illustrates the old way:
<div *ngIf="condition; else elseBlock">Content to render when condition is true.</div>
<ng-template #elseBlock>Content to render when condition is false.</ng-template>
To be honest, the new mode is easier for me to read.
@for block – repeaters
The @for repeatedly renders content of a block for each item in a collection. The collection can be represented as any JavaScript iterable but there are performance advantages of using a regular Array.
There are some variables available:
- $count: Number of items in a collection;
- $index: Index of current row;
- $first: Whether the current row is the first row;
- $last: Whether the current row is the last row;
- $even: Whether the current row index is even;
- $odd: Whether the current row index is odd.
Let’s see an example:
<div id="for">
<h2>For-Block</h2>
@for (item of items; track item.id; let idx = $index, e = $even, o = $odd, c = $count, f = $first, l = $last) {
<p>Item index: #{{ idx }} - Name: {{ item.name }}</p>
<p>Even: {{ e }} - Odd: {{ o }}</p>
<p>Count - Total items: {{ c }}</p>
<p>First: {{ f }} - Last: {{ l }}</p>
<hr/>
}
</div>
The value of the track
expression determines a key used to associate array items with the views in the DOM.
items = [
{
id: 1,
name: "Webcam"
},
{
id: 2,
name: "Mouse"
},
{
id: 3,
name: "Keyboard"
}
];
The result is:
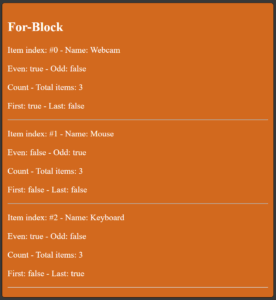
@empty block
You can use it after the @for; if the array is empty, it will display the content inside @empty, serving as a message for an empty array.
<div id="for">
<h2>Empty-Block</h2>
@for (item of items2; track item.name) {
<li> {{ item.name }} </li>
} @empty {
<li> There are no items. </li>
}
</div>
items2 = new Array;
The result is:
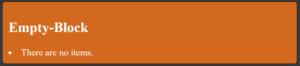
@switch block – selection
It is similar to the JavaScript switch, but there is no need for break or return. If no @case matches the expression and there is no @default block, nothing is shown.
<div id="switch">
<h2>Switch-Block</h2>
@switch (month) {
@case ('december') {
December.
}
@case ('november') {
November.
}
@default {
Default case.
}
}
</div>
month = "november";
The result is:
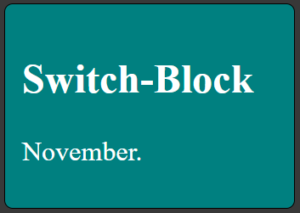
Conclusion
Angular 17 introduced several new features, and the new syntax is not only more readable but also comes with performance improvements behind the scenes. Don’t worry, though, because we can still use the old way; there’s no need to migrate if you prefer the existing approach.
Author
-
I am a seasoned Full Stack Software Developer with 8+ years of experience, including 6+ years specializing in Java with Spring and Quarkus. My core expertise lies in developing robust RESTful APIs integrated with Cosmos Db, MySQL, and cloud platforms like Azure and AWS. I have extensive experience designing and implementing microservices architectures, ensuring performance and reliability for high-traffic systems. In addition to backend development, I have experience with Angular to build user-friendly interfaces, leveraging my postgraduate degree in frontend web development to deliver seamless and responsive user experiences. My dedication to clean and secure code led me to present best practices to my company and clients, using tools like Sonar to ensure code quality and security. I am a critical thinker, problem solver, and team player, thriving in collaborative environments while tackling complex challenges. Beyond development, I share knowledge through my blog, NatanCode, where I write about Java, Spring, Quarkus, databases, and frontend development. My passion for learning and delivering innovative solutions drives me to excel in every project I undertake.