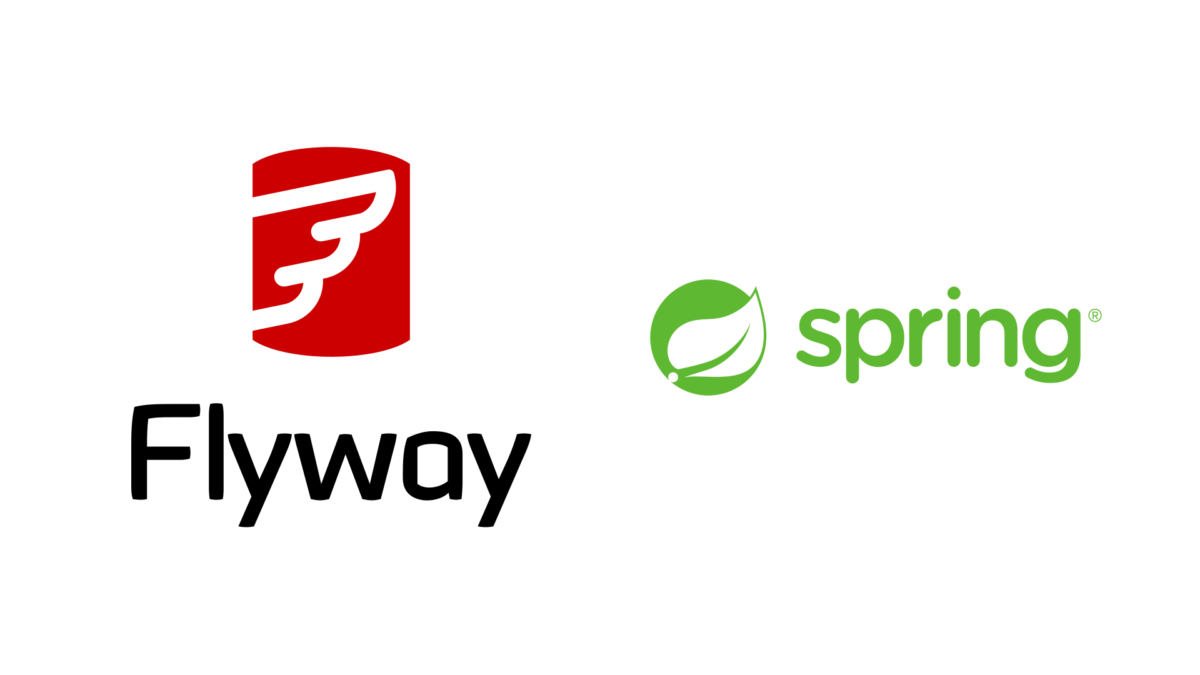
How to perform database schema versioning with Flyway and Spring?
Flyway is a tool used for database migration control. Migrations are executed automatically, and we also have a history of database modifications. Flyway supports many databases, but in this example, we will use MySQL. Requirements Project With this site, you can create your Spring project: https://start.spring.io To establish a connection with the database, we need…
Read More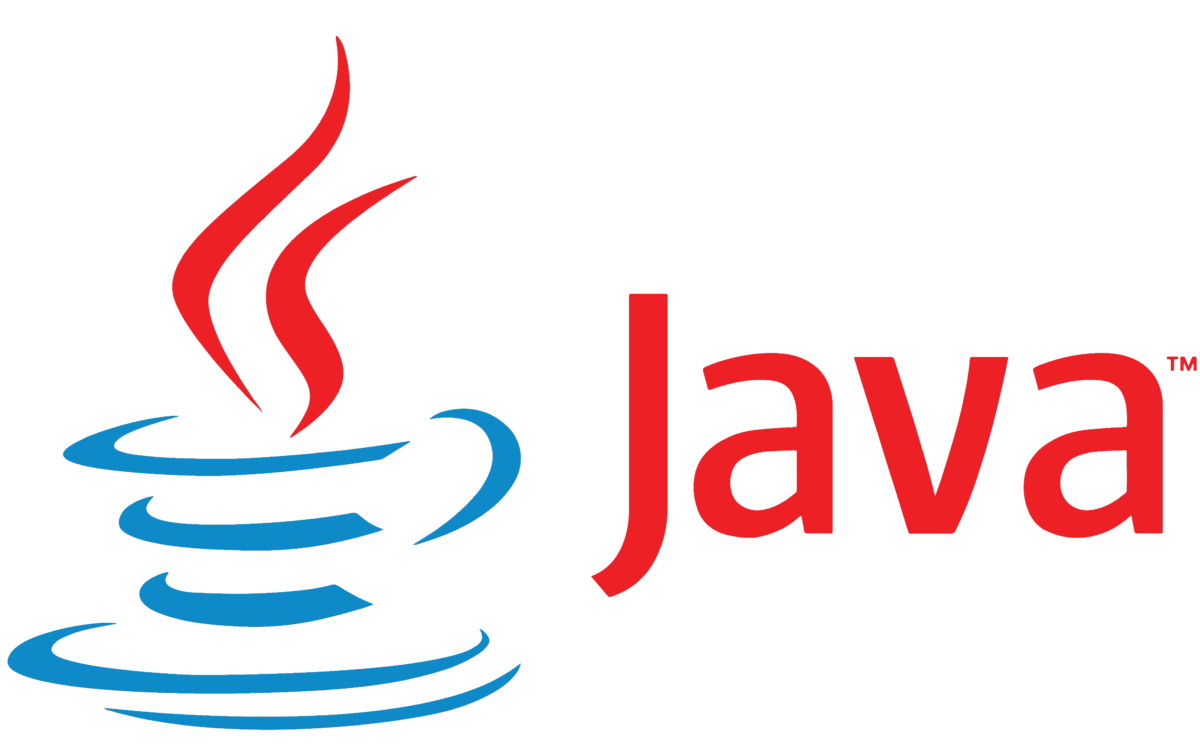
What is Garbage Collection in Java?
It is a process that manages memory in software written in Java. When running programs on the JVM (Java Virtual Machine), objects are created in the Heap memory. When these objects are no longer needed, the Garbage Collector will find unused objects and remove them from memory, helping to prevent memory leaks. We need to…
Read More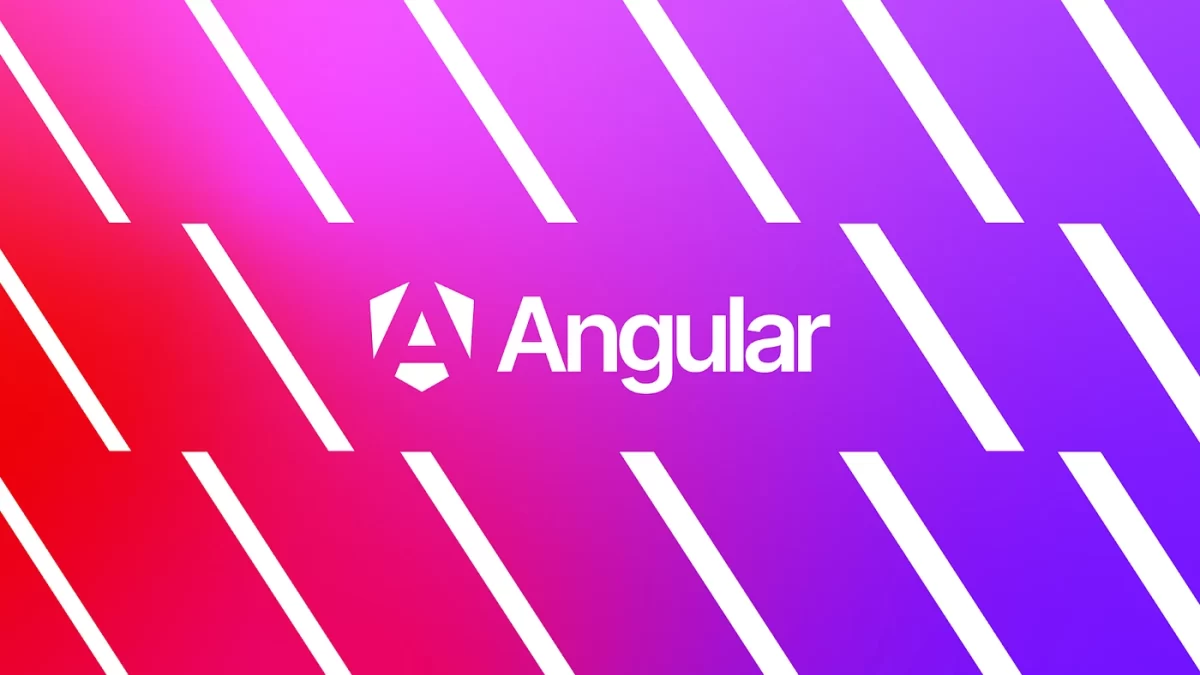
How to make custom directive in Angular?
Before creating a custom directive, we need to understand what a directive is in Angular. “Directives are classes that add additional behavior to elements in your Angular applications.” “Use Angular’s built-in directives to manage forms, lists, styles, and what users see.” The different types of Angular directives are as follows: Directive Types Details Components Used…
Read More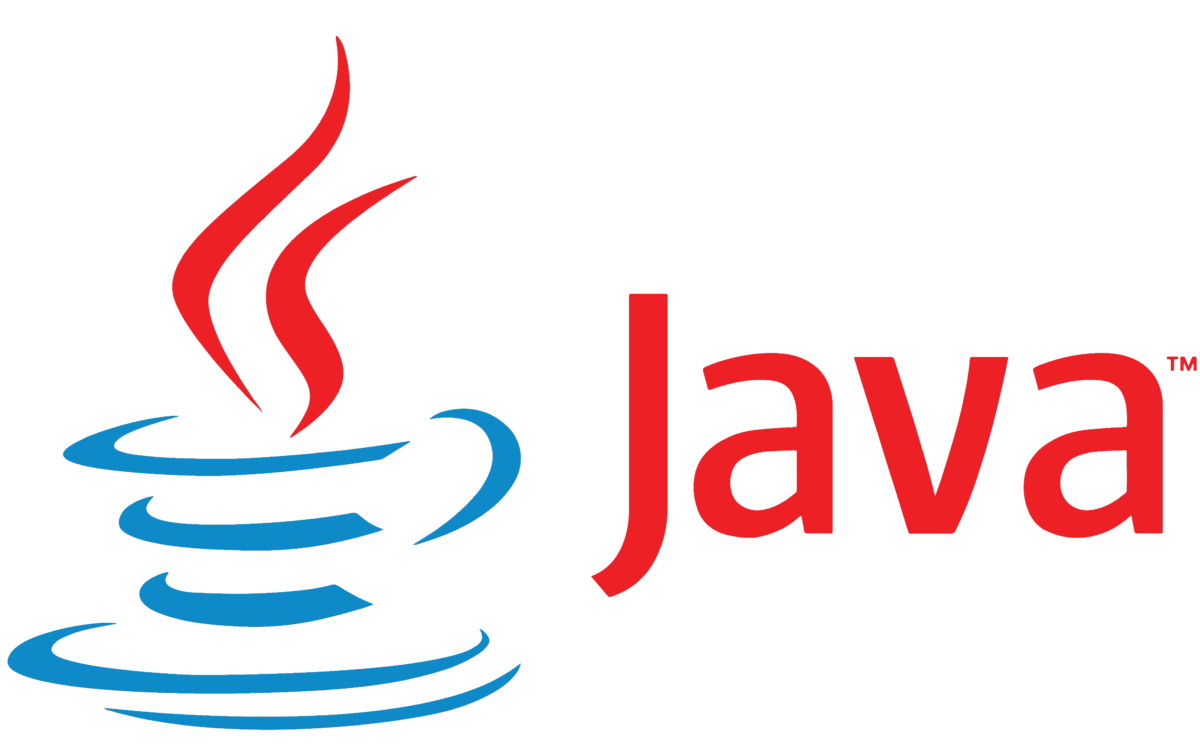
How to improve the performance of primitive types in Java’s Stream Api?
Java’s Stream API facilitates code maintenance, is used with lambda expressions and functional paradigm. It offers many features such as filtering a list, among others. Let’s see an example. For this, I created a class called Product. I populated a list of products and created the following stream. My goal is to map the quantity,…
Read More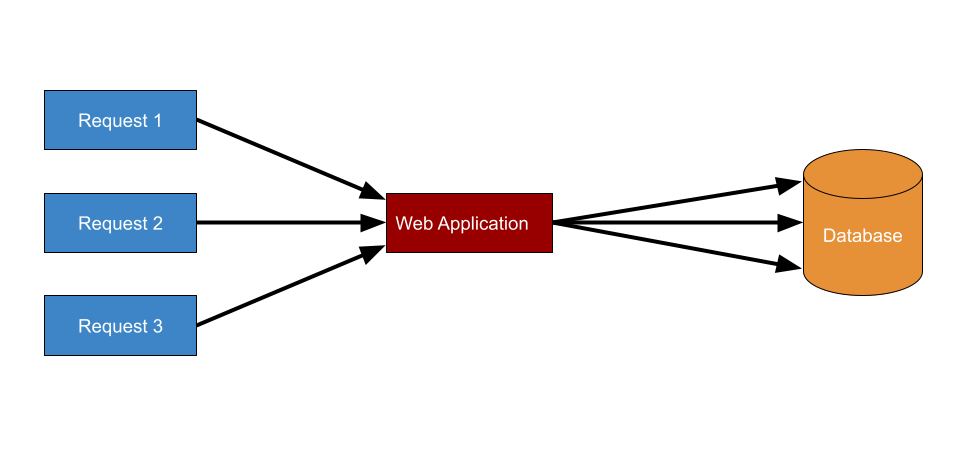
What is Connection Pool?
Connection Pool is very important for improving the response time of applications. To discuss connection pool, it’s important to understand how database connections work in an application. Let’s use the example of a web application, an API. Without Connection Pool A request was made to the application to fetch some information, and in this example,…
Read More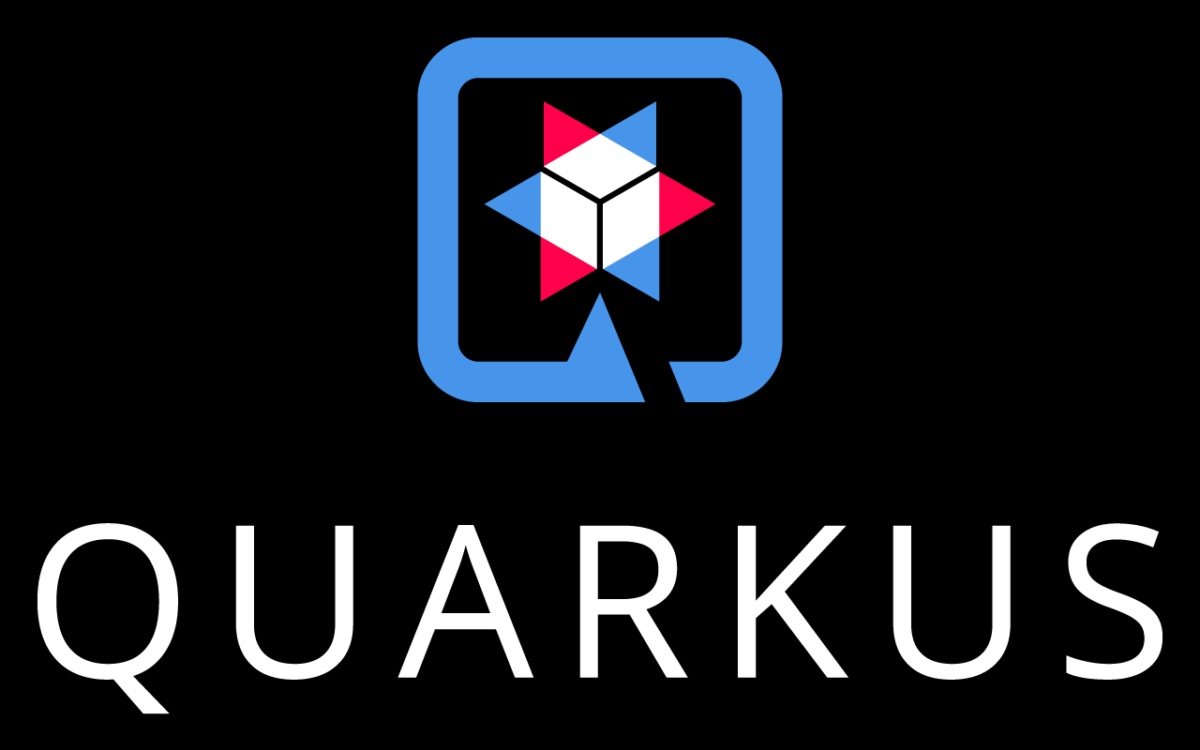
How to use Qualifier in Quarkus?
Imagine the following scenario where there are multiple implementations for the same interface, and when using dependency injection, the software needs to understand which implementation to use. Let’s illustrate with an example to understand what the problem is and how to solve it. Website Diagram: https://refactoring.guru/ In the example, we will have a delivery system that…
Read More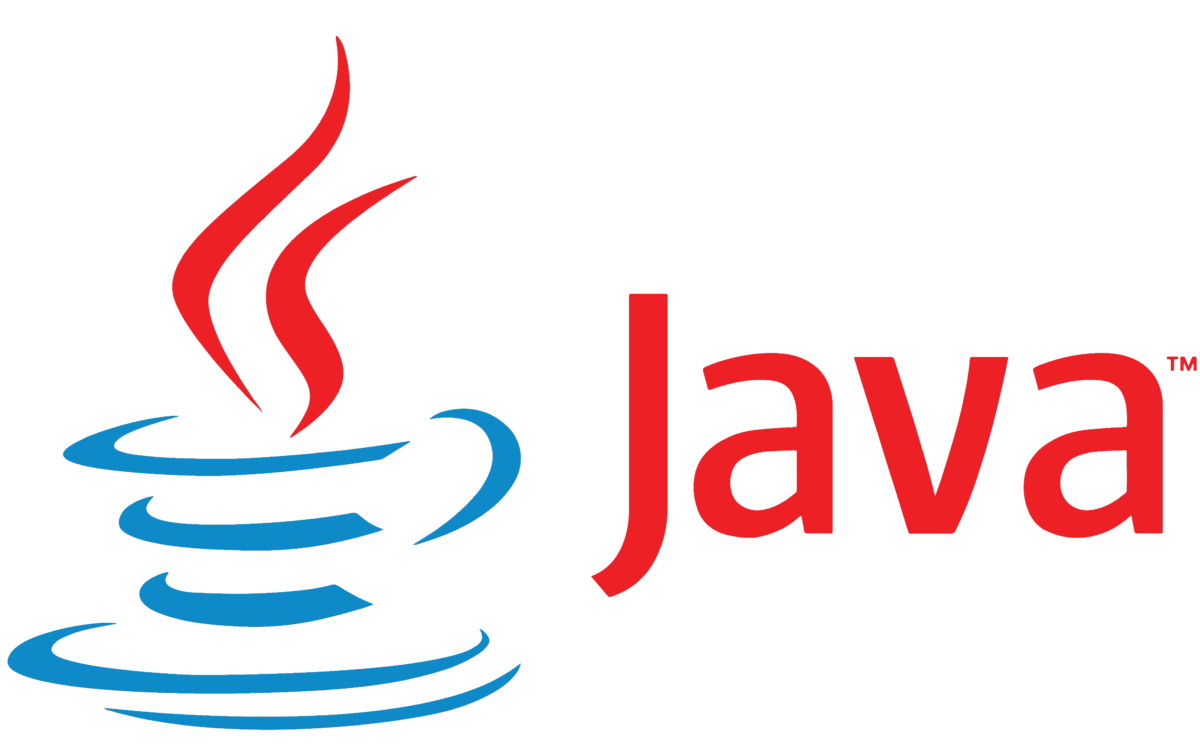
What is Dependency Injection?
To understand, I believe the best way is through an example. Let’s take a look at a simple delivery system. It’s not a complete system; it’s something didactic and simple to illustrate. Website Diagram: https://refactoring.guru/ In the example, we will have a delivery system that can be done by truck or ship, where there is…
Read More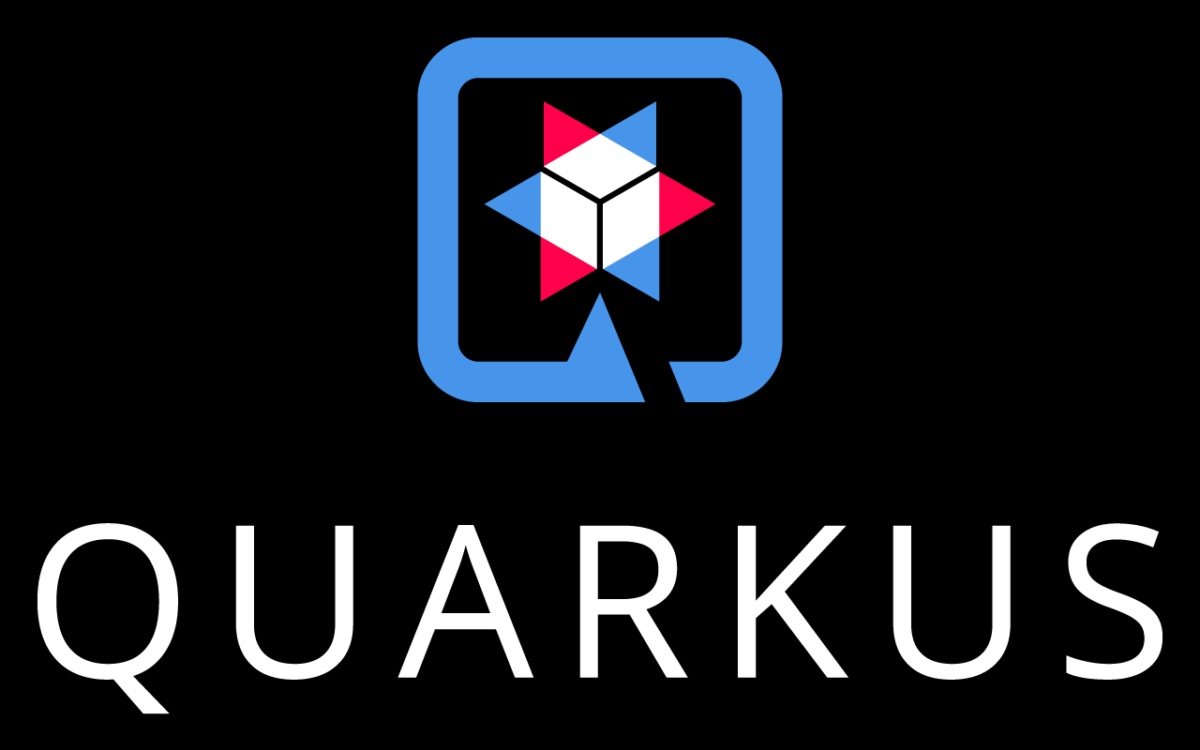
How to create a Custom Annotation in Quarkus Framework?
It’s important to perform validations in the system. There are dependencies available for this with a lot of ready-made solutions. For example, in the case of Quarkus, we can use Hibernate Validator, which provides us with some annotations to solve common problems. However, if it’s necessary to perform a validation for which there is no…
Read More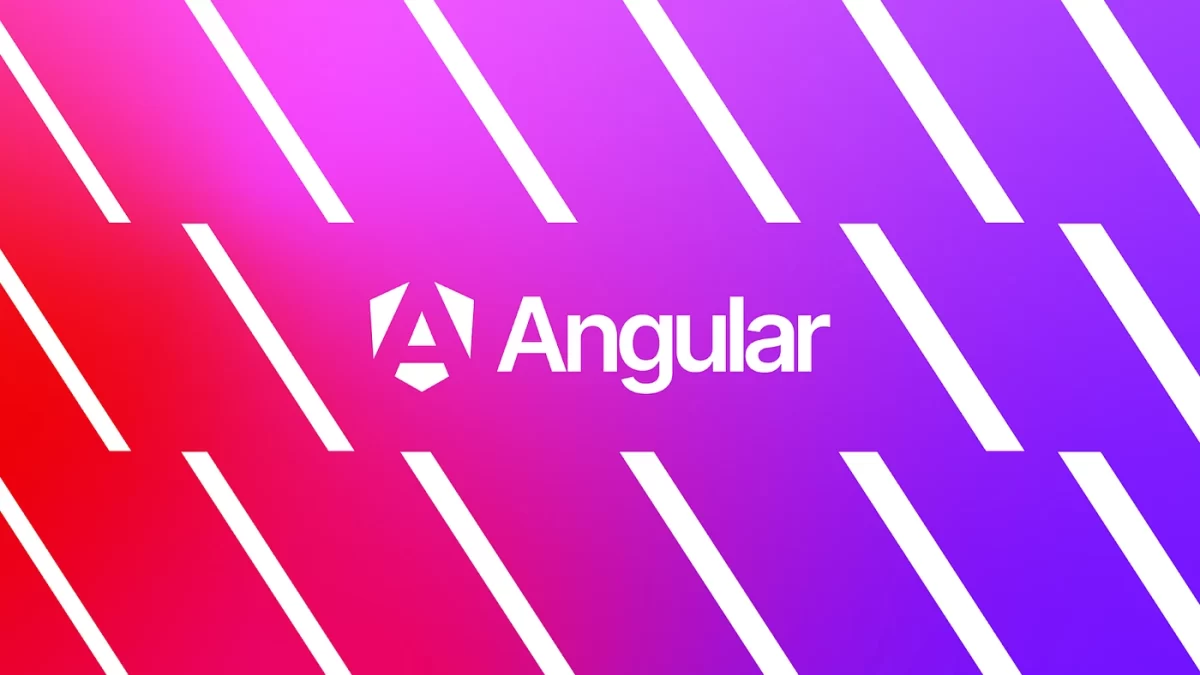
What is Data Binding in Angular?
It’s a way of communication between TypeScript code and HTML template. Let’s see an example below. I created a component called “user”. We have a variable that stores the value of the “username” and a method that resets this value, it’s a method triggered by a click event in the HTML template. In the HTML…
Read More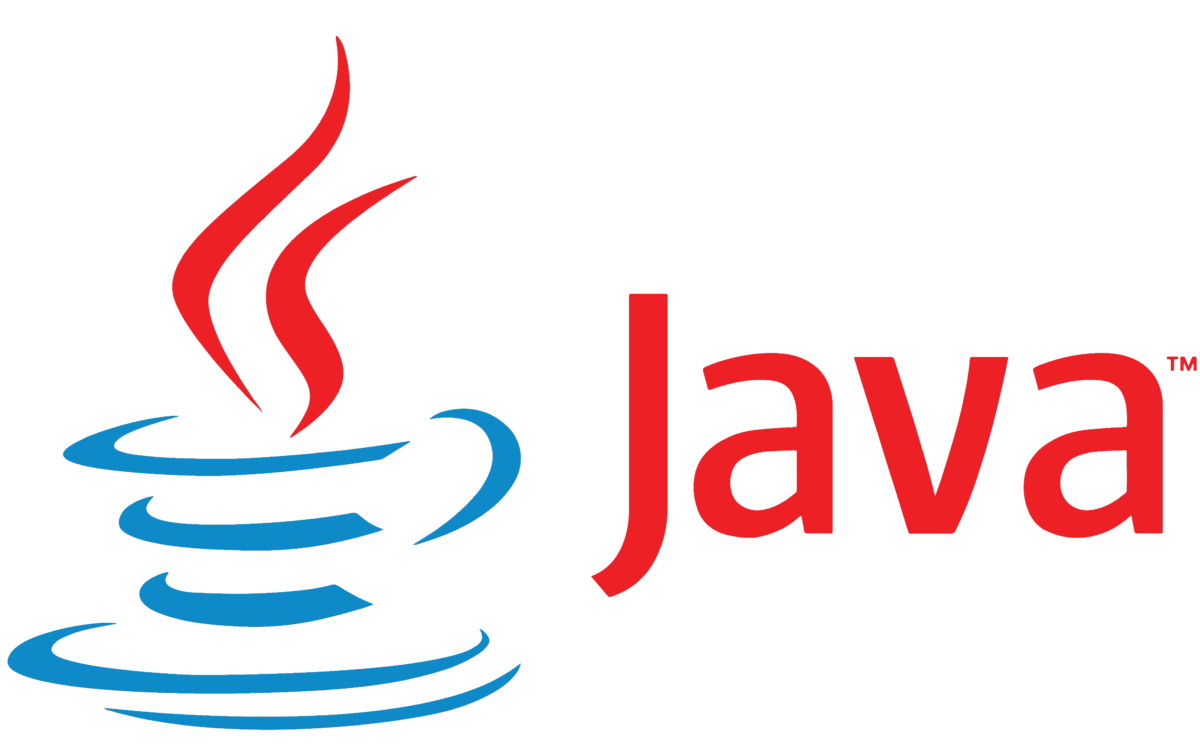
How does the Set interface work in Java?
The Set interface inherits from the Collection interface. There are several implementations of the Set interface, such as HashSet, LinkedHashSet, and TreeSet, each with its own characteristics. Unlike the List interface, we cannot access an element by index in a Set, but it is possible to iterate over the elements. We will see some usage…
Read More